Roblox Scripting 101: Meet Lua
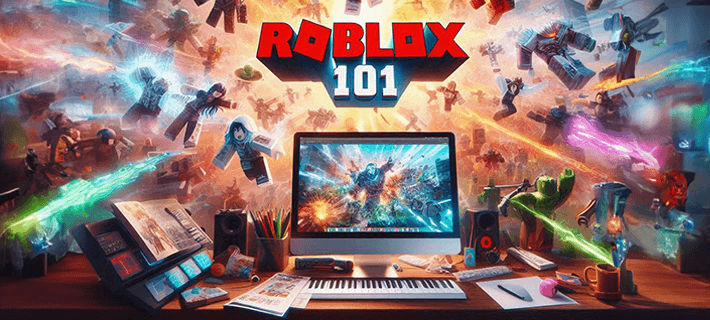
So, you want to be a Roblox developer, huh? Well, get ready to roll up your sleeves and dive into Roblox Scripting 101! We’ll take you through everything you need to know about Lua (don’t worry, it’s not as scary as it sounds) and how you can use it to make your Roblox games come alive. Ready? Let’s go! 👍
What is Roblox?⌗
Think of Roblox as a virtual playground where you not only play, but build your dream games. It’s where imagination meets code, and even if you’re just starting out, Roblox Studio (your toolkit) is designed to make game development fun and accessible. Whether you’re here to build an obstacle course that drives your friends mad or a massive multiplayer universe, you’ll need some scripting skills to really make things pop. Check out our Beginner’s Guide to Roblox Development for more on getting started.
Getting Started with Roblox Scripting⌗
First things first…
What is scripting? 🤔
Scripting means telling games how to behave, react, and interact. In Roblox we use Lua, an easy-to-learn, lightweight programming language.
-
Open up Roblox Studio > Explorer and hover over ServerScriptService to see the + button:
-
Click the + button and select Script
You now have a new script open in the editor 🎉
TIP: It’s a good idea to rename your script to something meaningful, like “HelloWorld”. As you get more scripts, you’ll want to keep them organised and decide on a naming convention that works for you.
Let’s get scripting… 💻
“Hello, World!” 👋⌗
One of the first things you learn in programming is how to print a message to the screen. Here’s how you’d do it in Lua:
print("Hello, World!")
Press Play in Roblox Studio and check the Output window to see your message (wave back if you like 🤣 ).
Comments: Keeping Notes in Your Code⌗
Comments are handy - especially when you’re learning, they can help you remember what you were doing and explain things to others. Here’s what comments look like in Lua:
-- This is a single-line comment (good for short notes & appending to code)
--[[
This is a multi-line comment.
You can write as much as you like here.
...and it won't affect your code.
]]
-- TODO: A todo comment is great for reminding yourself of things to do later
Variables: Storing Data⌗
What is a variable?: Variables are like containers that hold different types of information. You can change the value of a variable at any time. They’re super useful when your creating games.
Below you’ll find some examples of how to store different types of data in variables:
local a = 5 -- This is an integer (whole number)
local b = 3.142 -- This is a float (decimal number)
local c = "Roblox FTW!" -- This is a string (text value)
local d = true -- This is a boolean (true or false)
local e = nil -- This is nil (equal to nothing)
local f = {1, 2, 3} -- This is a table (a collection of strings, numbers or booleans)
Here’s a simple example of how you might use variables in a script to do some basic calculations:
-- Define some variables with integers
local x = 2
local y = 3
local z = 4
local xyz = x+y+z -- adding integers
local xLessY = x-y -- subtracting integers
local zTimeY = z*y -- multiplying integers
local zOverX = z/x -- dividing integers
-- Print the results
print("x + y + z = " .. xyz)
print("x - y = " .. xLessY)
print("z * y = " .. zTimeY)
You might also need to combine strings and variables together:
local player = "John"
local score = 0
--[[
when we adding two dots before or after a string
we can concatenate it with other variables
]]
print(player .. " has a score of " .. score)
Functions: Reusable Code Blocks⌗
Functions are like mini-programs within your script. They allow you to group code together and run it whenever you need. This avoids repeating the same code over and over and keeps your scripts neat and tidy.
Based on the calculations example above, you could create a function to add numbers:
local function AddNumbers(a:Number, b:Number)
return a + b
end
local result = AddNumbers(5, 10)
print("The result is: " .. result)
…or a function to greet a player:
-- Define a function called "greetPlayer"
local function GreetPlayer(playerName:String)
print("Hello, " .. playerName .. "!")
end
-- Call the function with a player name
greetPlayer("John")
Conditional Statements: Making Decisions⌗
Very often in games, you need to make decisions based on certain conditions. This is where if statements come in handy.
Here’s an example of an if statement that checks if a player’s score is greater than or equal to 100 & prints a different message based on the result:
local score = 100
if score >= 100 then
print("You're a winner!")
else
print("Try again!")
end
And you can also use else if to check multiple conditions:
local score = 50
if score >= 100 then
print("You're a winner!")
elseif score >= 50 then
print("So close, keep going...")
else
print("Try again!")
end
Loops: Repeating Code⌗
Loops are used to repeat a block of code multiple times. There are two main types of loops in Lua: for and while.
Here’s an example of a for loop that prints numbers from 1 to 5:
for i = 1, 5 do
print(i)
end
And here’s an example of a while loop that prints numbers from 1 to 5:
local i = 1
while i <= 5 do
print(i)
i = i + 1
end
Depending on what you’re trying to achieve, you might use one loop over the other. For example, if you know how many times you want to repeat a block of code, you might use a for loop. If you’re not sure how many times you want to repeat a block of code, you might use a while loop.
Did you know? You can also use break to exit a loop early, or continue to skip the rest of the loop and move to the next iteration.
Here’s an example of a for loop that breaks early:
for i = 1, 10 do
if i == 5 then
break
end
print(i)
end
…and the same using a while loop:
local i = 1
while i <= 10 do
if i == 5 then
break
end
print(i)
i = i + 1
end
Tables: Organizing Data⌗
Tables, also known as arrays or lists, are used to store multiple values in a single variable. They’re super useful for storing data like player names, scores, or game objects.
Here’s an example of a table that stores player names:
local players = {"John", "Jane", "Alice", "Bob"}
-- Loop through the table and print each player's name
for i, player in ipairs(players) do
print("Player " .. i .. ": " .. player)
end
If you need to store more complex data, you can use key-value pairs in a table:
local myTable = {
["name"] = "John",
["age"] = 30,
["city"] = "New York"
}
for key, value in ipairs(myTable) do
print(key, value)
end
Error Handling: Dealing with Mistakes⌗
Scripting is awesome, but it doesn’t always go smoothly 😠
When you run into errors, don’t panic! Roblox Studio has a built-in Output window that shows you what went wrong. Here are a few common errors you might see:
- Syntax Error: This means you’ve made a mistake in your code. Check your spelling, punctuation, and make sure you’re using the right commands.
- Nil Value: This means you’re trying to use a variable that doesn’t exist. Check your variables and make sure they’re defined and spelt correctly.
TIP: If you’re stuck, don’t be afraid to ask for help. The Roblox community is full of friendly developers who are happy to lend a hand. You can also check out the Roblox Creator Hub for more resources and tutorials.
Remember: Your code will throw an errors, and very often you’ll have no idea why. But don’t sweat it! 😅 Debugging is part of the fun.
Conclusion⌗
And that’s a wrap on Roblox Scripting 101! 🙌 Whether you’re building a small Obby or an RPG masterpiece, mastering the art of scripting is the key to success. Remember, game development is all about experimenting and learning, so don’t be afraid to break things and try again. When in doubt, hit up our Beginner’s Guide to Roblox Development for more tips, tricks, and inspiration.
Now get out there and make something amazing! 🕹️ 🎉
Related Posts
- Beginner’s Guide to Roblox Development
- Easy Rainbow Obby! The Alpha Release
- Fire and Ice: Creating Particle Effects in Roblox
- Top 5 Most Popular Roblox Game Genres
- Halloween (v1.0)